Standard cross sectionΒΆ
[1]:
from shape_generator import CrossSectionHolding
[2]:
unit = 'cm'
height = 200
width = 100
label = 'test'
r_channel (float)
r_roof (float)
r_wall (float)
slope_bench (float)
r_round (float)
r_wall_bottom (float)
h_bench (float)
pre_bench (float)
w_channel (float)
Dictionary """""""""" +---------+---------------------+--------------------------+ | english | deutsch | description | +=========+=====================+==========================+ | channel | Trockenwetter-Rinne | | +---------+---------------------+--------------------------+ | roof | Firste/Decke | Bogen an der Rohr Firste | +---------+---------------------+--------------------------+ | wall | Wand | Radius der Seitenwand | +---------+---------------------+--------------------------+ | bench | Berme | Neigung um der TW-Rinne | +---------+---------------------+--------------------------+[3]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit)
[4]:
import pandas as pd
pd.DataFrame(cs.get_points()).T.rename(columns={0: '$h_i$', 1: '$w_i$'})
[4]:
$h_i$ | $w_i$ | |
---|---|---|
0 | 0.0 | 0.0 |
1 | 0.0 | 50.0 |
2 | 200.0 | 50.0 |
3 | 200.0 | 0.0 |
[5]:
fig = cs.profile_figure()
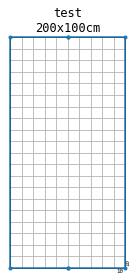
[6]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_channel=35)
fig = cs.profile_figure()
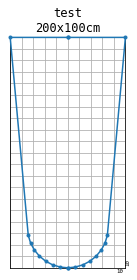
[7]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_channel=35,
pre_bench=45)
fig = cs.profile_figure()
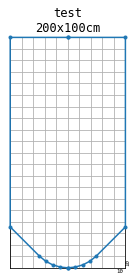
[8]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_channel=35,
pre_bench=45,
slope_bench=5)
fig = cs.profile_figure()
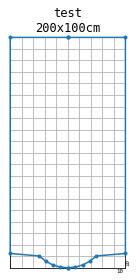
[9]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_channel=35,
h_bench=25,
slope_bench=5)
fig = cs.profile_figure()
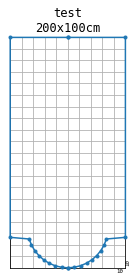
[10]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_channel=35,
w_channel=25,
slope_bench=5)
fig = cs.profile_figure()
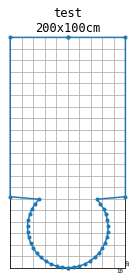
[11]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
slope_bench=15)
fig = cs.profile_figure()
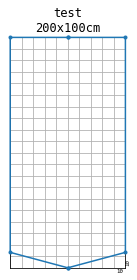
[12]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_channel=30,
slope_bench=15)
fig = cs.profile_figure()
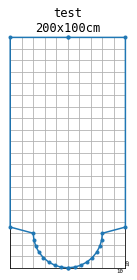
[13]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_roof=100)
fig = cs.profile_figure()
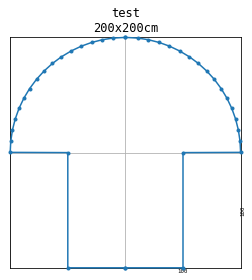
[14]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_roof=30, r_wall=110)
fig = cs.profile_figure()
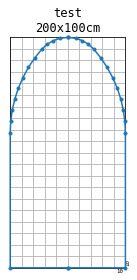
[15]:
cs = CrossSectionHolding.standard(label, height=height, width=width, unit=unit,
r_roof=20)
fig = cs.profile_figure()
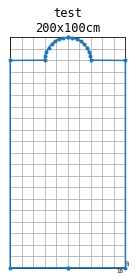
You can even make a standard egg cross section
[16]:
r = 30.
cs = CrossSectionHolding.standard(label, height=r * 3, width=r * 2, unit=unit,
r_channel=r / 2, r_wall=3 * r, r_wall_bottom=3 * r, r_roof=r, h_bench=r / 5)
fig = cs.profile_figure()
/home/markus/PycharmProjects/SWMM_xsections_shape_generator/shape_generator/helpers.py:131: UserWarning: unused part of the shape detected. Ignoring this part.
warnings.warn('unused part of the shape detected. Ignoring this part.')
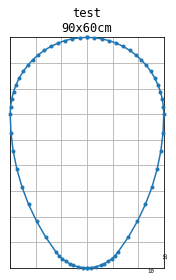
The warning occurred because the r_wall part was ignored. However, this input cannot be omitted, otherwise the following will happen.
[17]:
r = 30.
cs = CrossSectionHolding.standard(label, height=r * 3, width=r * 2, unit=unit,
r_channel=r / 2,
r_wall=3 * r,
r_roof=r,
h_bench=r / 5)
fig = cs.profile_figure()
/home/markus/PycharmProjects/SWMM_xsections_shape_generator/shape_generator/helpers.py:131: UserWarning: unused part of the shape detected. Ignoring this part.
warnings.warn('unused part of the shape detected. Ignoring this part.')
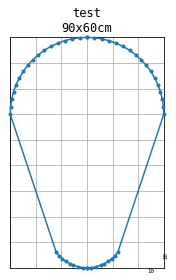
[18]:
r = 30.
cs = CrossSectionHolding.standard(label, height=r * 3, width=r * 2, unit=unit,
r_channel=r / 2,
r_wall_bottom=3 * r,
r_roof=r,
h_bench=r / 5)
fig = cs.profile_figure()
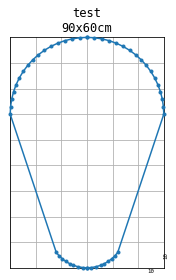